Cache, Queue & Session Management with Redis
Redis is a powerful in-memory data structure store serving as a database, cache, message broker, and queue. This guide walks you through Redis integration with your Laravel application on Zerops for high-performance caching, session management, and queue processing.
Zerops provides Valkey, an open-source Redis alternative that delivers high-performance key/value storage with full Redis compatibility. Valkey supports all common Redis use cases — from caching and message queues to primary database functionality.
Adding Redis Service​
To use Valkey (Redis) features with Laravel, first either import Valkey as a service to your Zerops project
or add the Valkey service to your project manually from the Zerops GUI.
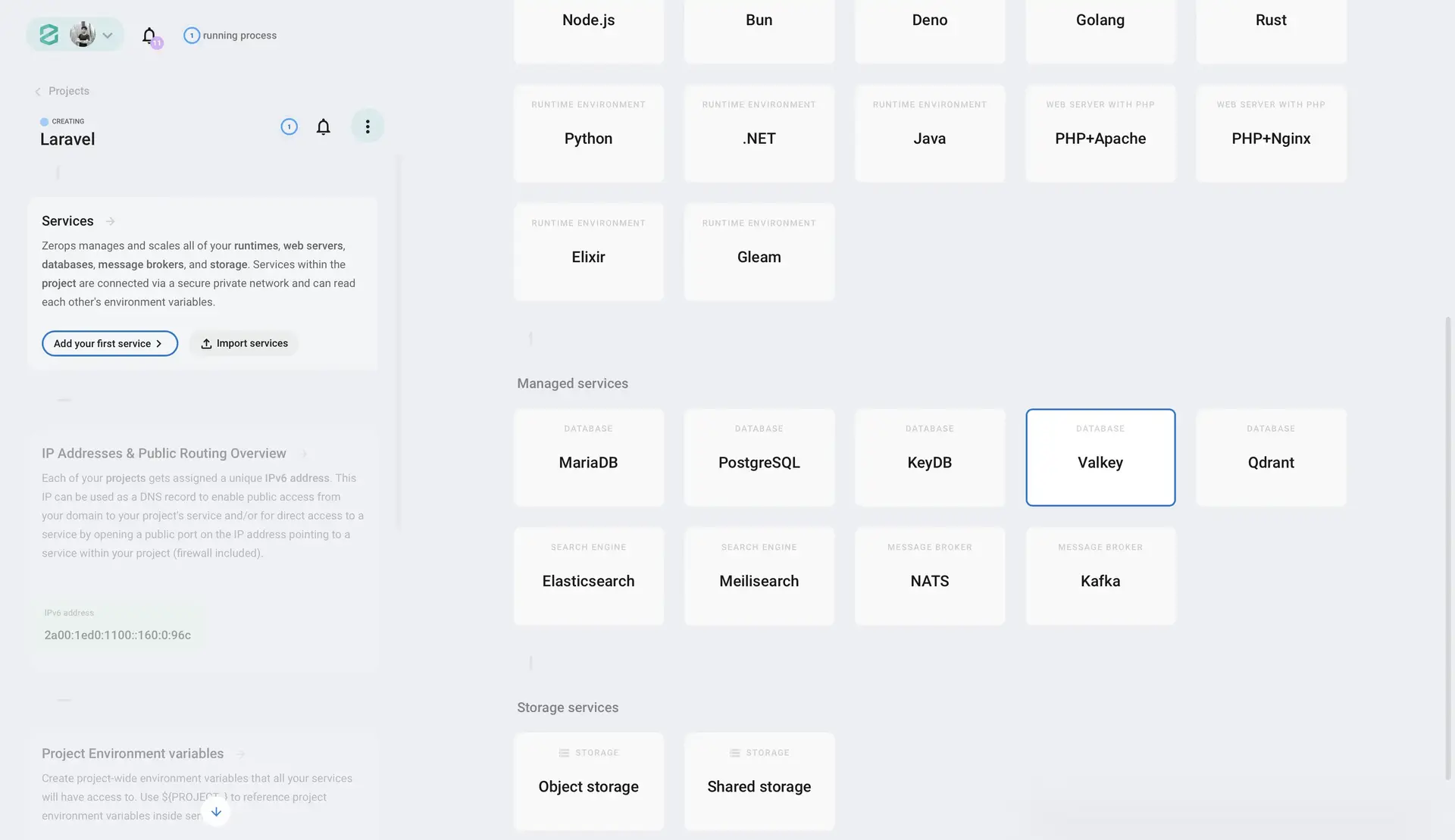
For production environments, we recommend using HA
mode. This configuration:
- Ensures automatic failover during node failures
- Provides data replication across multiple nodes
- Improves reliability and uptime
Environment Configuration​
Basic Redis Settings​
Environment variables control how your Laravel application connects to and uses Redis. Below are the core settings grouped by functionality:
zerops:
- setup: app
build:
base:
- php@8.4
os: alpine
run:
base: php-nginx@8.4
os: alpine
siteConfigPath: site.conf.tmpl
envVariables:
# Redis Connection Settings
REDIS_CLIENT: phpredis # PHP Redis client for better performance
REDIS_HOST: valkey # Internal hostname of Valkey service
REDIS_PORT: 6379 # Standard Redis port number
# Cache Configuration
CACHE_PREFIX: cache # Namespace for cache keys
CACHE_STORE: redis # Use Redis as primary cache
# Session Configuration
SESSION_DRIVER: redis # Store sessions in Redis
SESSION_ENCRYPT: false # Disable session encryption
SESSION_LIFETIME: 120 # Session timeout in minutes
SESSION_PATH: / # Cookie path setting
# Queue Configuration
QUEUE_CONNECTION: redis # Use Redis for job queues
BROADCAST_CONNECTION: redis # Redis for event broadcasting
Feature-Specific Configuration​
Redis Caching​
Laravel's cache system offers a unified API across different cache backends. The following configuration establishes Redis as your primary cache store for fast, reliable data caching:
'default' => env('CACHE_STORE', 'database'),
'stores' => [
'redis' => [
'driver' => 'redis',
'connection' => env('REDIS_CACHE_CONNECTION', 'cache'),
'lock_connection' => env('REDIS_CACHE_LOCK_CONNECTION', 'default'),
],
],
'prefix' => env('CACHE_PREFIX', Str::slug(env('APP_NAME', 'laravel'), '_').'_cache_'),
Session Management with Redis​
Using Redis for session storage provides better performance than file-based sessions and enables seamless session sharing across multiple application servers. You can also set up a custom session connection in the config/session.php
file.
Queue System Configuration​
Redis queues offer a robust solution for handling background jobs in Laravel. This configuration sets up Redis as your queue backend with retry policies and connection settings. You can also set up a custom queue connection in the config/queue.php
file.
Consider using Supervisor for managing Laravel queues in production.
Performance Optimization​
Configure your Redis connection pool for optimal performance: